Sokoban
16 Jun 2022 - Java
Sokoban is a 80’s game where the objetive is to put every boxes of the grid into the goal points to pass to the next level. In this version we change every game sprites to make it prittier and different.
Controls
The controls are very simple. There are only 4 keys to play, each for every cardinal directions:
- W Up
- A Left
- S Right
- D Down
Shortcuts
Apart of the existing buttons in the GUI, there are available shortcuts to make the gameplay more dynamic and easy.
- Ctrl+Z Undo the movement
- Ctrl+Shift+Z Redo the movement
- Ctrl+S Save the current game
- Ctrl+L Load a game
- Ctrl+N New game
Demo
Figbot
21 Apr 2022 - Java
Figbot is an intelligent twitch bot which helps the streamer to moderate the chat. The proupose of this project was to use jade, a java library for an agent oriented paradigm. Every agent has a unique responsability and they communicate with to others by TCP/IP messages.
Commands
Figbot includes a set of commands where the user can type them in the chat with the prefix f!
:
f!help
f!greetings [user]
f!shoutout [user]
f!dice [sides]
f!time
f!video [user]
f!title
Figbot is able to ban or timeout, among others, automatically recognising intelligently the message of the user who don’t behave in a polite way.
Interface
The app incorporates a GUI where are displayed the most importants moderation events like:
- Slow and slow off the chat
- Deleted messages
- Timeouts
- Bans and unbans
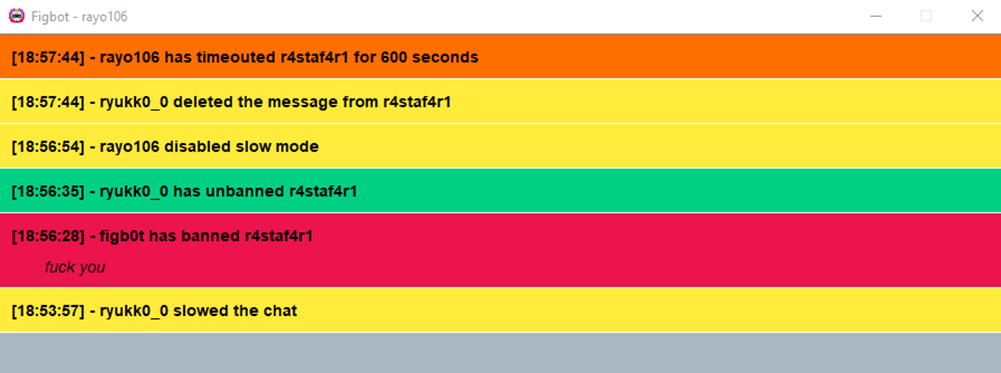
On the twitch side we can see every moderation events in the chat. Here are presented some examples:
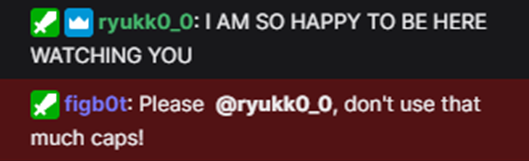
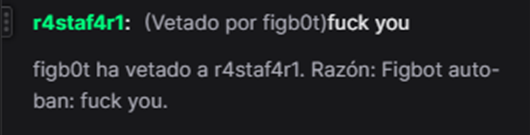
Javascript Processor
13 Jan 2022 - Java
This is one of my biggest projects I have ever done. It is a reduced lexic, syntactic and semantic processor of a modified version of javascript where you give it a source javascript file and it returns if the file is well written. In case of failure, it will give you the lines where the error is and the mistakes you did, like a usual compiler.
Symbols
There are a few logic and aritmethic symbols that I implemented:
<
Minor than
!
Negation
-
Minus
=
Assignment
%=
Modular assignment
Apart of this, thare are the essential symbols like round and curly brackets, double slash for commenting lines, single quotes for string variables and so on.
Variables, functions, decisions and loops
Here we declare some of this modified javascript language types, predefined functions, statements and functions.
int
, boolean
, string
print
, input
if
, for
function
Examples
This is a correct input:
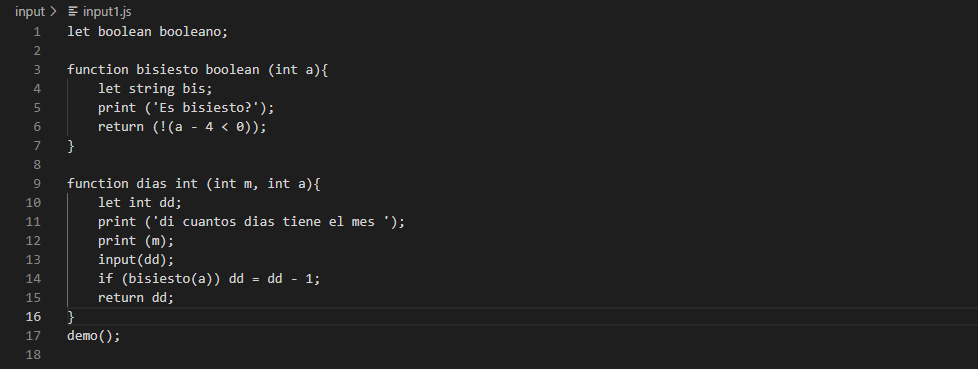
So the processor prints out that everything is good:

This is an incorrect input:
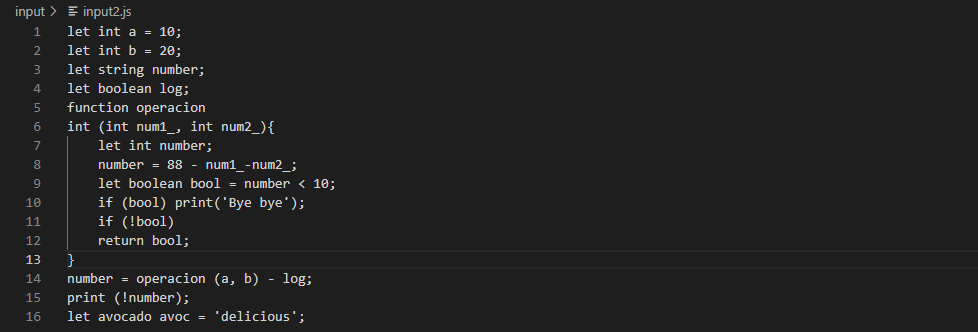
And the processor says that we have to check a file where the errors are displayed:

The errors file:

Minishell
22 Dec 2021 - C
This project is one of the most satisfying things I could ever done. I always was very
curious on how a shell works internally and this project gives me the first idea. This shell is a minimal version of a real big shell like bash or zsh for example. It was made in C by using multiple processes and executions (fork() and exec()).
Internal commands
The shell is completly ready to execute internal commands of the OS like:
ls
date
cat
echo
And an infinite list of commands ...
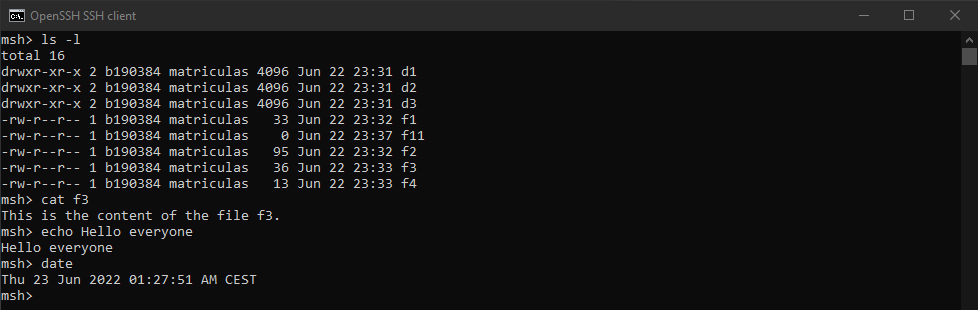
External commands
There are also another commands that are commitment of the own shell. I implemented this:
cd [dir]
umask [value]
limit [resource [max]]
set [variable [value...]]
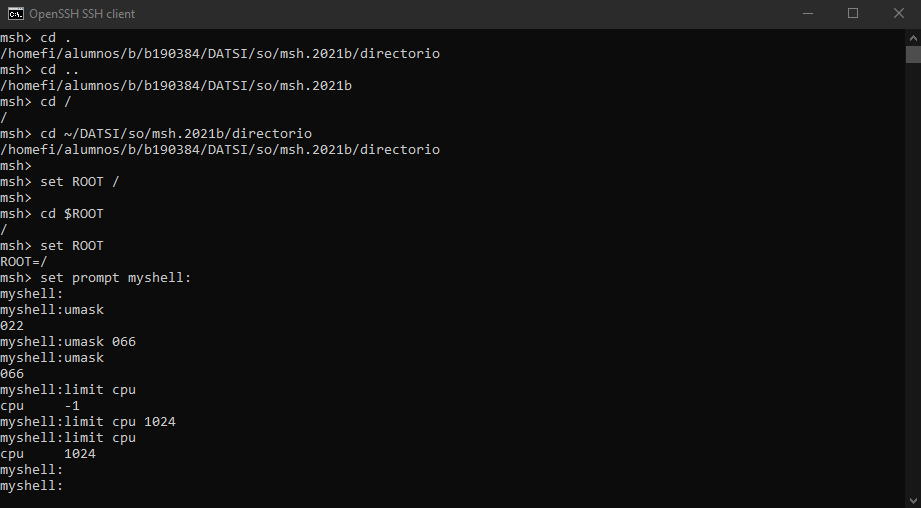
Pipes, redirections, background and signals
-
As we all know, we can use pipes A | B
to use the standard output of A
as the standard input of B
. Each pipe creates a new child that is responsible of executing its command with the standard output of the left child.
-
We can also redirect the standard output to a file with A > file
or the redirect the standard input from a file with A < file
.
-
If we want to send to the background some task we can do it by typing &
at the end of the command. Example: sleep 10 &
.
-
On the one hand we can’t kill the shell with Ctrl+C or Ctrl+\ it will be impossible, like a real shell. The only way of exiting the shell is by inserting a EOF with Ctrl+D. On the other hand we can kill processes that we executed in the foreground or background.
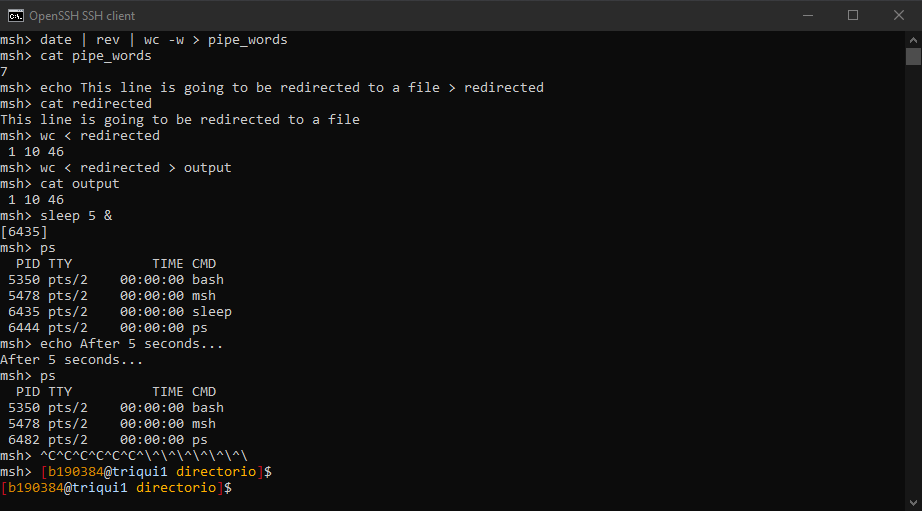
-
The shell incorporates the posibility of the using the ? wildcard, which tries to replace a character in that position.
- The shell interpretates some special characters like ~ or $
~[user]
if user appears, then is replaced by the home
of user
. Otherwise by the home’s user that executed the command.
$variable
replace it by the value
of the environment variable variable
.
- There are special variables that the shell interpretes in a special way.
prompt
The minishell prompt (msh> by default)
mypid
The pid of the shell process
bgpid
The pid of the last ran background process
status
The return value of the last command or pipeline
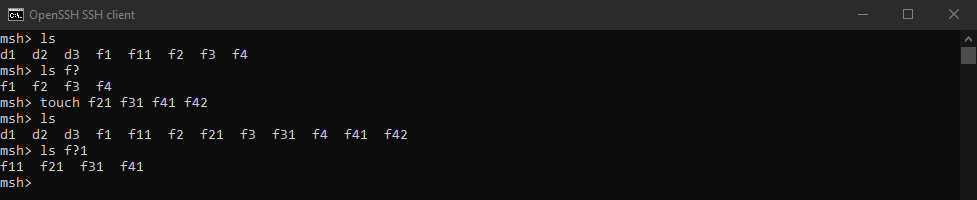
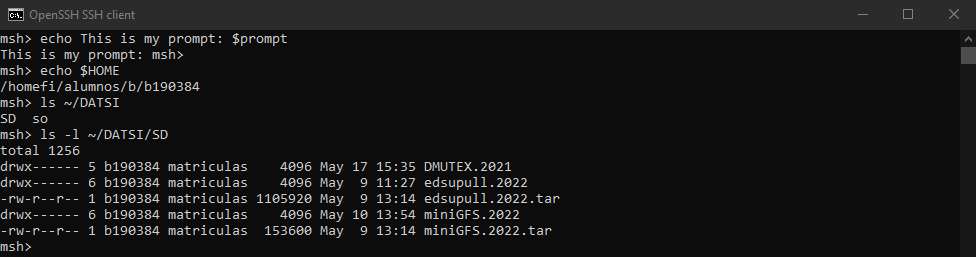
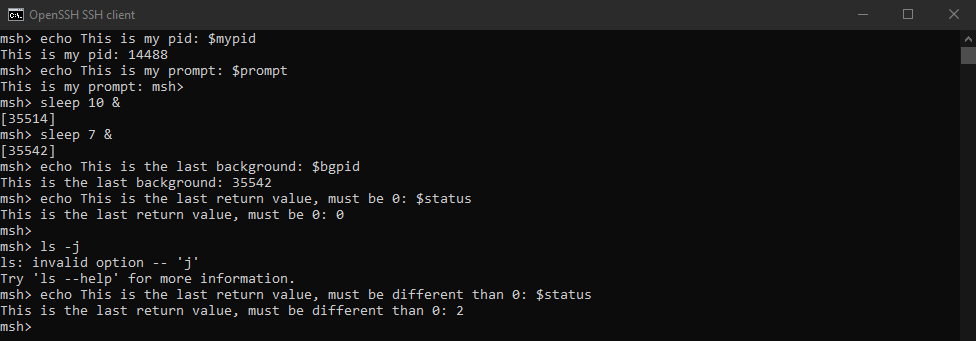
Game of life
02 Nov 2021 - Python
The game of Life is a cellular automaton designed by John Horton Conway in the 70’s.
There are no players in this game, just an initial state. The game consists on a board with multpile cellules, which can be alive or dead. The initial state determines which cells are alive and dead.
There are only 3 rules in this game:
- Any live cell with two or three live neighbours survives.
- Any dead cell with three live neighbours becomes a live cell.
- All other live cells die in the next generation. Similarly, all other dead cells stay dead.
Only with this 3 rules we can observe a lot of interesting patterns.
Examples
Here is an infinite loop which generetes a lot of ‘spacecraft’
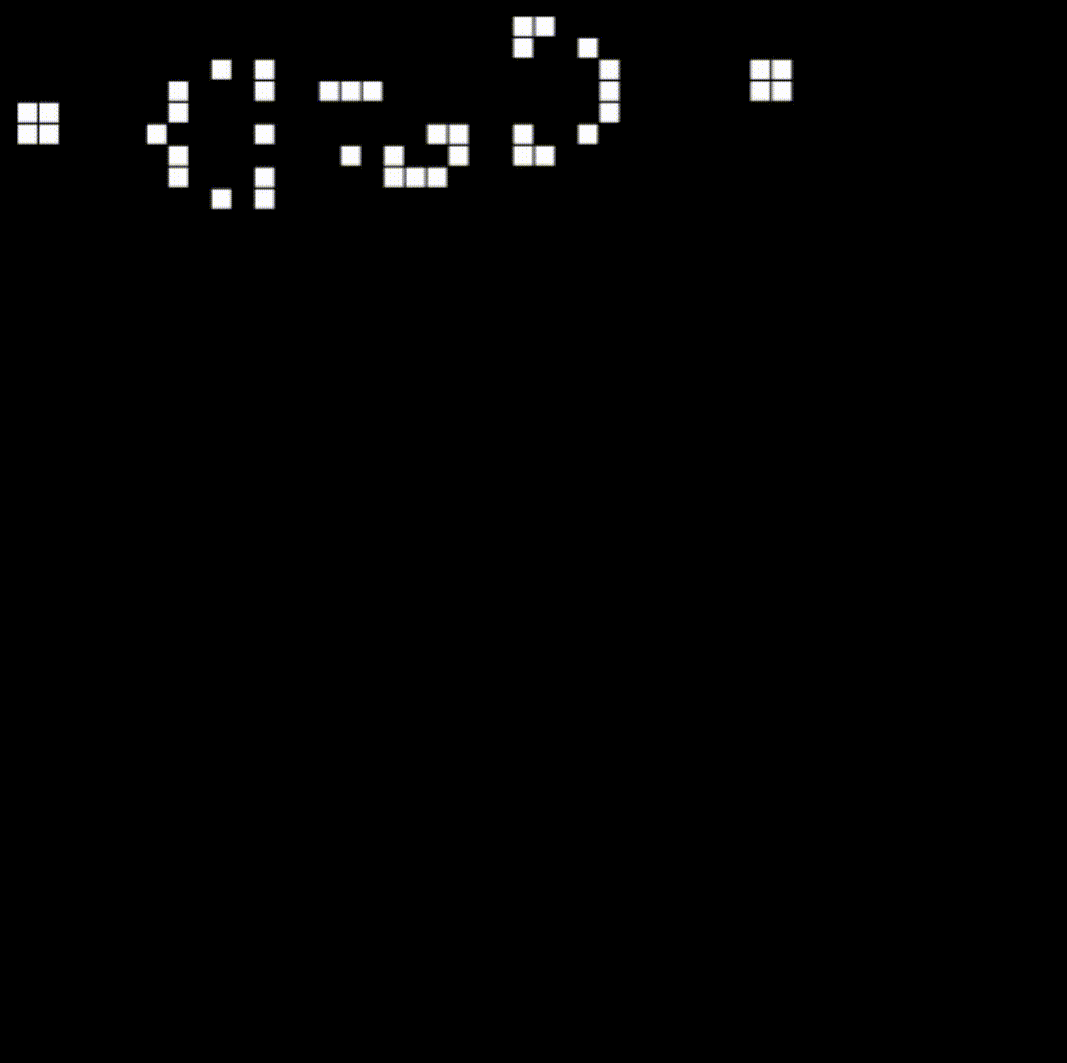